# IMDB Clone React Website ## Project Description We will be creating an IMDB clone, where we will fetch Real-time trending movies data and will show it in grid format, we will be designing the whole application by using Tailwind CSS. ## Features of the project The following will be the features of our IMDB clone: - The user will be able to view all the latest & trneding movies (IMDB API) - User can create his own separate watchlist - User can filter movies according to genre - User can sort movies according to ratings - Pagination will be implemented to move from one page to another to get updated data of other movies - Search feature will be there for movies. - We will be deploying this to netlify ## Wireframe 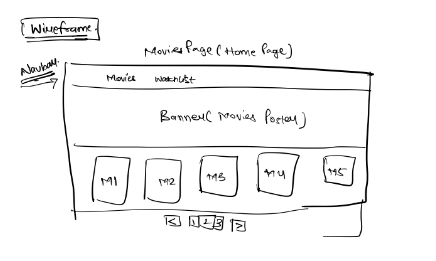 ## Implementation > **Note**: This lecture is in continuation to *'Full Stack LLD & Projects: React-4: IMDB Project- Part 1'*, please refer that lecture before hopping onto this one. In this lecture we will be implementing the following features: - Briefing Client side Routing and React Router - Watchlist Component - Pagination ### Component - **WatchList.jsx** - This JavaScript file defines the `WatchList` component, which represents the user's watchlist section in the IMDb web application. Users can view and manage the list of movies they have added to their watchlist. - The component function takes several props: - `watchList`: An array containing the user's watchlist of movies. - `handleRemoveFromWatchList`: A function to handle the removal of movies from the watchlist. - `setWatchList`: A function to update the watchlist state. - The component also uses local state variables to manage filtering and searching of watchlist movies. These state variables include `genreList`, `currGenre`, and `search`. - Inside the component, there are several functions and effects that manage various aspects of the watchlist: - `hanldeFilter(genre)`: Updates the current genre filter when a genre is clicked. - `handleSearch(e)`: Updates the search query when the user types in the search input. - `sortIncreasing()`: Sorts the watchlist in increasing order of movie ratings. - `sortDecreasing()`: Sorts the watchlist in decreasing order of movie ratings. - The `useEffect` hook is used to populate the `genreList` state with unique genres based on the movies in the watchlist. It ensures that the genre filter options are updated whenever the watchlist changes. - The component's `return` statement defines the structure of the WatchList component: - Genre filter buttons are displayed at the top. Users can click on these buttons to filter the watchlist by genre. - A search input field allows users to search for movies in the watchlist based on their titles. - A table displays the watchlist movies, including columns for movie name, ratings, popularity, genre, and a delete option. - The table rows are generated based on the current genre filter and search query. - The ratings column headers allow users to sort the watchlist in ascending or descending order based on movie ratings. - The delete option in each row allows users to remove a movie from their watchlist. - The `WatchList` component provides essential functionality for users to manage and view their watchlist, making it a valuable feature of the IMDb web application. **Code**: ```javascript= import { useEffect, useState } from "react"; import genreids from "../Utility/genre"; function WatchList(props){ let {watchList,handleRemoveFromWatchList,setWatchList} = props; let [genreList,setGenreList] = useState(["All Genres"]); let [currGenre,setCurrGenre] = useState("All Genres"); let [search,setSearch] = useState(""); let hanldeFilter = (genre)=>{ setCurrGenre(genre) } let handleSearch = (e)=>{ setSearch(e.target.value); } let sortIncreasing = ()=>{ let sorted = watchList.sort((movieA,movieB)=>{ return movieA.vote_average-movieB.vote_average }) setWatchList([...sorted]); } let sortDecreasing = ()=>{ let sorted = watchList.sort((movieA,movieB)=>{ return movieB.vote_average-movieA.vote_average }) setWatchList([...sorted]); } useEffect(()=>{ let temp = watchList.map((movieObj)=>{ return genreids[movieObj.genre_ids[0]]; }) temp = new Set(temp); setGenreList(["All Genres",...temp]); },[watchList]) return( <>
Name |
Ratings
|
Popularity | Genre | |
---|---|---|---|---|
{movieObj.title}
|
{movieObj.vote_average} | {movieObj.popularity} | {genreids[movieObj.genre_ids[0]]} | handleRemoveFromWatchList(movieObj)} className=" text-red-600">Delete |